Add a network
In some cases, such as when interacting with smart contracts,
your dapp must connect a user to a new network in MetaMask.
Instead of the user adding a new network manually,
which requires them to configure RPC URLs and chain IDs, your dapp can use the
wallet_addEthereumChain
and
wallet_switchEthereumChain
RPC methods to prompt
the user to add a specific, pre-configured network to their MetaMask wallet.
These methods are specified by EIP-3085 and EIP-3326, and we recommend using them together.
wallet_addEthereumChain
creates a confirmation asking the user to add the specified network to MetaMask.wallet_switchEthereumChain
creates a confirmation asking the user to switch to the specified network.
The confirmations look like the following:
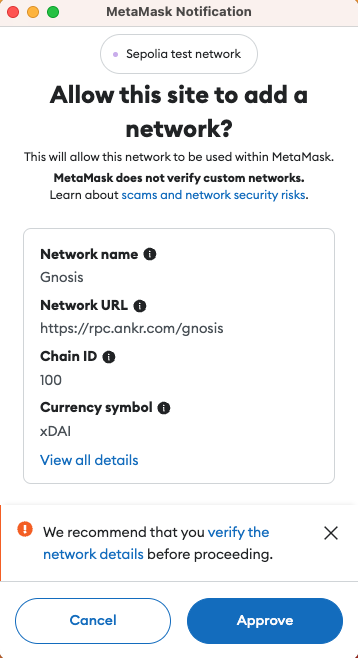
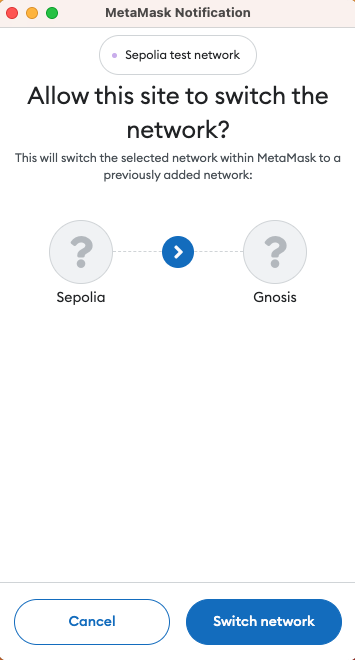
Development and non-EVM networks
- To add a local development network such as Hardhat to MetaMask, see Run a development network.
- To add a non-EVM network such as Starknet to MetaMask, see Use non-EVM networks.
Example
The following is an example of using wallet_addEthereumChain
and wallet_switchEthereumChain
to
prompt a user to add and switch to a new network:
try {
await provider // Or window.ethereum if you don't support EIP-6963.
.request({
method: "wallet_switchEthereumChain",
params: [{ chainId: "0xf00" }],
})
} catch (switchError) {
// This error code indicates that the chain has not been added to MetaMask.
if (switchError.code === 4902) {
try {
await provider // Or window.ethereum if you don't support EIP-6963.
.request({
method: "wallet_addEthereumChain",
params: [
{
chainId: "0xf00",
chainName: "...",
rpcUrls: ["https://..."] /* ... */,
},
],
})
} catch (addError) {
// Handle "add" error.
}
}
// Handle other "switch" errors.
}